Industrial Control Technology Sharing Platform
>
PLC_OPEN is a standard in the field of industrial automation programming. Codesys is a software system platform developed by the German company 3S, which fully supports the PLC_OPEN standard (specifically the IEC61131-3 standard). It supports six PLC programming languages: IL, ST, FBD, LD, CFC, and SFC. Users can choose different languages to edit subroutines, function modules, etc., within the same project.
Many companies currently develop their automation controllers based on this platform, such as Schneider, Beckhoff, ABB, IFM, Bosch Rexroth, and HollySys, among others.
Using Codesys programming to implement the Modbus Tcp Server function facilitates communication between controllers with Ethernet interfaces and HMIs or PCs for customers. It reduces customer costs and enhances the flexibility of the system’s use.
Introduction to Modbus Tcp Application Layer Protocol and Data Frame
Modbus-TCP has become one of the open internet standards today and is recognized by the Internet Engineering Task Force (IETF), an organization for internet standards. As part of the Modbus protocol remains unchanged, the well-known Modbus service and object model remain valid, with the only change being the porting of its transport layer protocol to TCP/IP.
The Modbus Tcp application layer protocol is independent of the communication medium and organized based on the client/server model. A client sends a request frame to ask for service, and the server responds with a response frame. The request and response frames contain parameters and/or data. Figure 1 shows the standard Modbus Tcp frame format. In standard Modbus communication, the slave address and CRC check are prioritized over the function code. However, in Modbus Tcp, the address and check are completed by the underlying Tcp protocol.
There are structural differences between Modbus Tcp and Modbus_RTU in data datagrams, where the message frame header replaces the Slave ID with the MBAP.
The MBAP consists of 7 bytes and appears at the head of each Modbus Tcp information frame. These 7 bytes comprise the Transaction Identifier (2 bytes), Protocol Identifier (2 bytes), Length (2 bytes), and Unit Identifier (1 byte, device station number).
>
Figure 1: Using Modbus-TCP, commands and user data can be encapsulated in a TCP/IP data container without any modification.
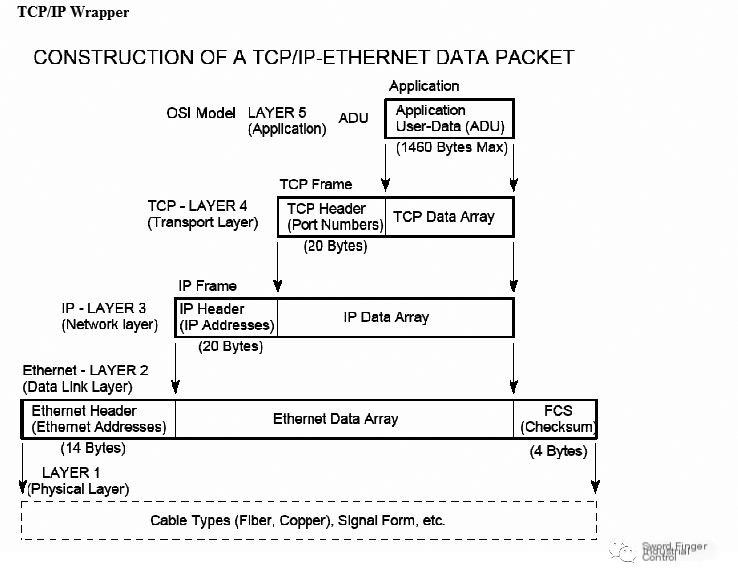
Figure 2: Structure after Ethernet TCP/IP protocol encapsulates Modbus-TCP ADU
1. Hardware and Software Configuration for Implementing MODBUS_TCP SERVER
1.1 The project selects LTI company’s MOTION ONE PAC controller
(Model: LACP242, INTEL_ATOM CPU, 1.1GHZ)
Hardware Resources: ETHERNET 10/100M interface, CODESYS2.3 software platform (Figure 3)
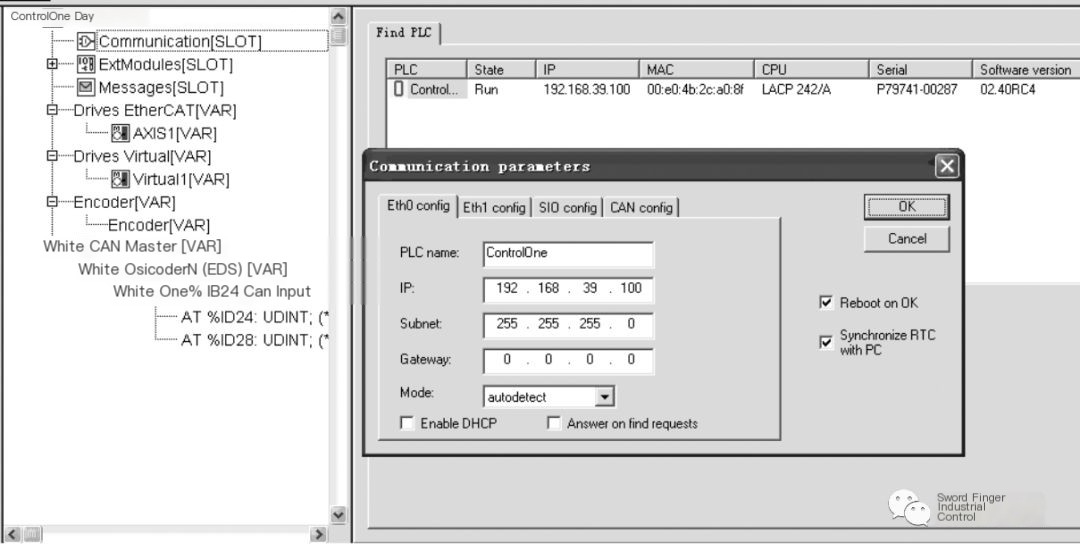
Figure 3: Configuration platform for Codesys2.3 software
1.2 Select the TCP/IP communication library function SyslibSockets.lib from the 3S company’s CODESYS2.3 software platform, while mainly using the following functional functions:
- SysSockInetAddr(ip): Binds the IP address of the specified Ethernet interface, the IP address of the Ethernet port on the controller itself
- SysSockHtons(port): Binds the Ethernet port, MODBUS_TCP usually uses port 502
- SysSockCreate(SOCKET_AF_INET, SOCKET_STREAM, 0): Creation of Tcp/IP Socket
- SysSockBind(socketId, ADR(), SIZEOF()): Tcp/IP Socket binds to the specified port and IP address
- SysSockListen(socketId, 255): Tcp/IP Socket performs port listening
- SysSockSelect(SOCKET_FD_SETSIZE, ADR(), 0, 0, ADR()): Selecting TCP/IP client
- SysSockAccept(socketId, ADR(), ADR()): TCP/IP Socket accepts client device connections
- SysSockRecv(SocketHandle, ADR(), SIZEOF(), 1): TCP/IP Socket receives data packets from client devices
- SysSockSend(SocketHandle, ADR(), (), 1): TCP/IP Socket returns data packets, sending them to the Client device
- SysSockClose(socketId): Close TCP/IP Socket.
1.3 The Process of Creating a MODBUS_TCP SERVER
The process of creating a MODBUS_TCP server is mainly divided into the following steps:
1.3.1 Installation of the TCP/IP Socket Library File
Add the SyslibSockets.lib under the library manager column in the CODESYS2.3 software platform. You can then call the Ethernet communication function normally.
1.3.2 Declare various communication parameter variables and data structures, such as the MODBUS_TCP SERVER IP address and port, and the array for data sending and receiving:
Var_Global
addressPointer: POINTER TO SOCKADDRESS;
address: SOCKADDRESS;
ip: STRING := ‘192.168.39.100’; (*Controller’s IP address*)
……
port: WORD := 502; (*MODBUS-TCP port*)
objectArray: ARRAY[0..6] OF REAL;
tcp_connect_state: BOOL; (*TCP/IP connection status word*)
protocol_id: INT; (*Protocol identifier ID, modbus id = 00 00*)
device_id: INT; (*Device station number ID*)
END_VAR
1.3.3 Description of MODBUS_TCP SERVER Main Program.
The main program structure diagram and part of the program is as follows:
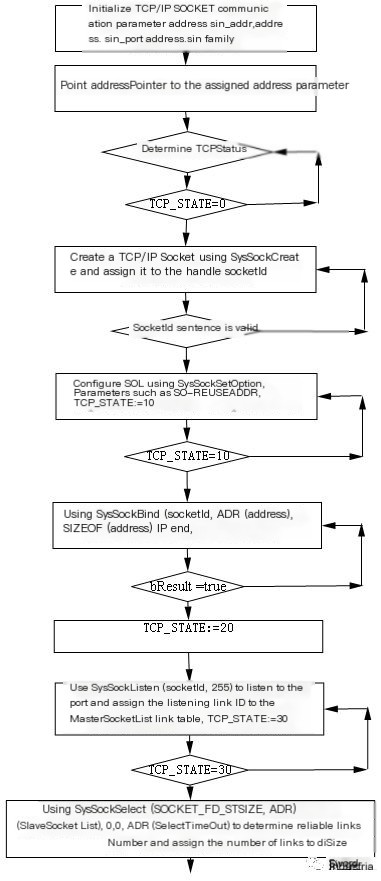
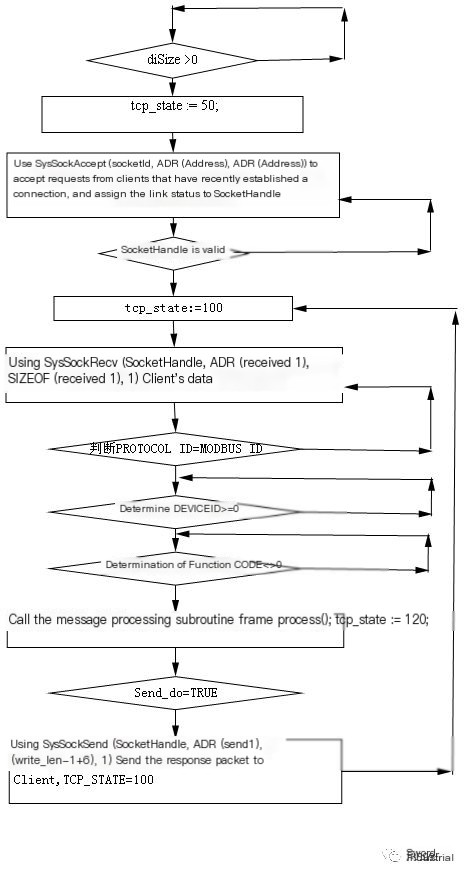
The main program completes port binding by calling the Sockets function within the SyslibSockets.lib library and implements monitoring of the corresponding port. When a Modbus Tcp client requests a connection, the server establishes the connection and exchanges read and write data. To facilitate judgment of the connection status between the client and server, the program performs real-time message refresh. After establishing a communication connection, if no new messages are received by the server and a period is maintained, it will process the communication interruption, close Sockets, reinitialize parameters, and the server will reenter listening status. This control mode can identify situations such as network physical disconnection and abnormal client disconnection.
address.sin_addr := SysSockInetAddr(ip);
IF terminate=FALSE THEN
CASE tcp_state OF
0:
socketId := SysSockCreate(SOCKET_AF_INET, SOCKET_STREAM, 0);
IF socketId <> SOCKET_INVALID THEN
SysSockSetOption(socketId, SOCKET_SOL, SOCKET_SO_REUSEADDR, ADR(dwValue), SIZEOF(dwValue));
tcp_state := 10;
END_IF
10: bResult := SysSockBind(socketId, ADR(address), SIZEOF(address));
IF bResult THEN
tcp_state := 20;
END_IF
……
50:
SocketHandle := SlaveSocketList.fd_array[TCPindex];
IF SocketHandle = socketId THEN
diSize := SIZEOF(Address);
SocketHandle := SysSockAccept(socketId, ADR(Address), ADR(address));
tcp_state := 100;
END_IF
100:
(* SysSockSend(socketId, ADR(send), SIZEOF(send), 1); *)
SysSockRecv(SocketHandle, ADR(receive1), SIZEOF(receive1), 1);
……
IF protocol_id=0 AND device_id>=0 AND receive1[1]<>0 THEN (*modbus_tcp, protocol_id=0*)
frame_process();
tcp_state := 120;
END_IF;
END_CASE;
END_IF;
1.3.4 Creating MODBUS_TCP SERVER Message Processing Program (Partial Subroutine)
After the server receives a message from the client, upon validating the Modbus Tcp protocol ID and function code’s effectiveness, it calls the message processing program. In this program, handling is done according to the function codes 01, 02, 03, 04, 05, 06, 15, 16 for MODBU-TCP read and write word, read and write bit functions, respectively. For example, when processing the write register function, it first determines the starting address of the register to be written and the number of registers to be written, then performs address offset assignment. High- and low-byte conversions are performed during the assignment process to ensure data correctness.
Frame_process (*MODBUS_TCP message processing*)
IF (send_do = FALSE) THEN
CASE input_byte1[2] OF
03: (*Read register*)
……
address_temp := SHL(BYTE_TO_INT(input_byte1[3]), 8) + (BYTE_TO_INT(input_byte1[4]));
……
FOR move_to_send := address_temp TO address_temp + (length_temp) * 2 DO
output_byte[4 + move_to_send – address_temp] := mw_area[move_to_send + address_temp + 1];
END_FOR;
……
END_CASE;
END_IF;
2. Validation and Use of MODBUS_TCP server
Once the MODBUS_TCP server communication program is complete, the MODBUS-TCP message is analyzed using the Wireshark Ethernet packet capture software, and MODBUS_TCP communication between the touchscreen and PAC controller was achieved using the Easybuilder800 touchscreen software.
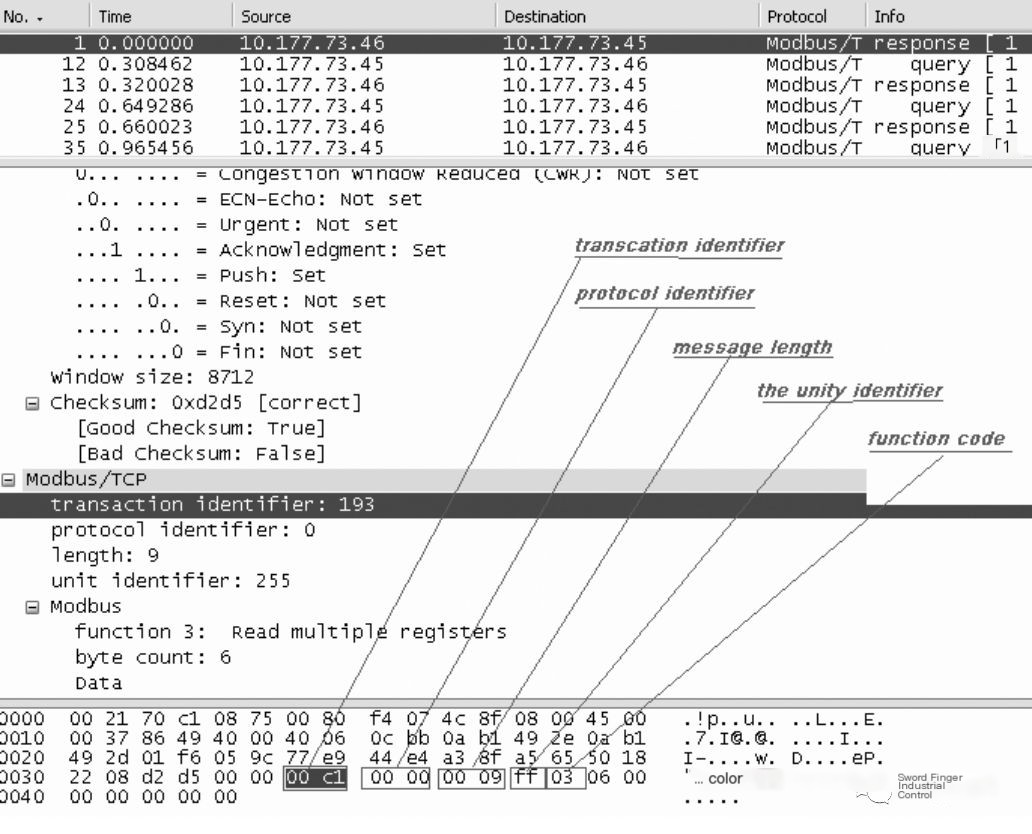
Figure 4: MODBUS-TCP Messages Analyzed by Wireshark Ethernet Packet Capture Software
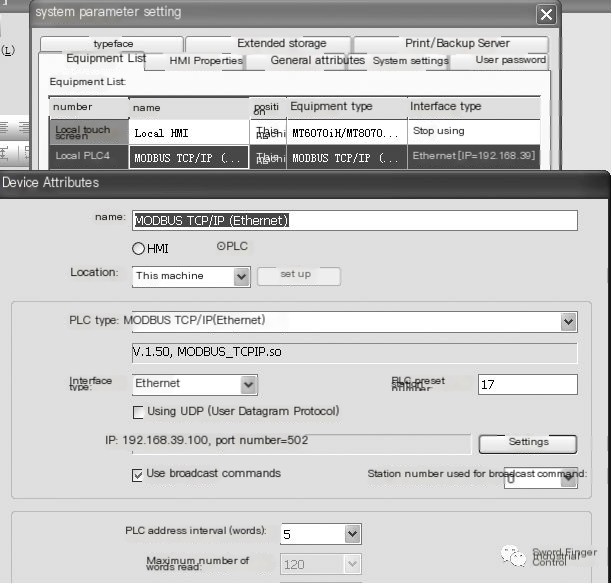
Figure 5: Configuring Communication Interface with Easybuilder800 Touchscreen Software
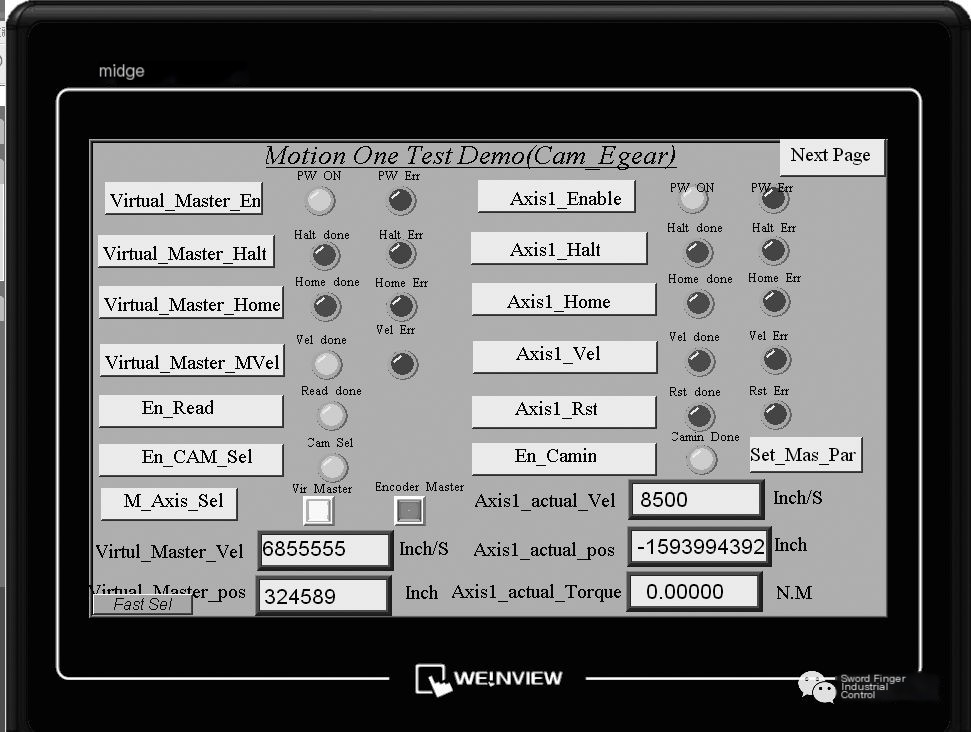
Figure 6: Monitoring State of Controller Variables with Easybuilder800 Touchscreen Software
Conclusion
Programming with sockets on the Codesys software platform allows PAC controllers to communicate with any Ethernet device using a public protocol. The functions of the library functions inside Codesys are rich, and the flexibility of PAC controller communication programming is more powerful than that of PLC.
Program Configuration and Source Code
Implementation of MODBUS TCP SERVER on LTI MOTION ONE Controller (Codesys)
I. Brief Function Overview:
Due to customer cost requirements, customers want to choose a third-party touchscreen to communicate with LTI’s MOTION ONE (Ethernet). The communication interface chosen is the Ethernet interface on the controller body. Because the MODBUS TCP SERVER communication software function block is not a built-in block of the CODESYS kernel, it needs to be purchased separately, so I developed a MODBUS TCP SERVER DEMO program using the SYSockets.LIB built-in function block inside CODESYS. This way, MOTION ONE can communicate over Ethernet with almost all touchscreens on the market.
II. Program Configuration and Source Code:
This program’s function is verified through actual MODBU-TCP communication with MODSCAN and Weilun touchscreen. This program supports the 01, 03, 05, 06, 15, and 16 MODBU-TCP read and write word, and read and write bit function codes.