ICMP Protocol
Common header
0 1 2 4
0 1 2 3 4 5 6 7 8 0 1 2 3 4 5 6 7 8 9 0 1 2 3 4 5 6 7 8 0 1 2 3 4
+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+
| Type | Code | Checksum |
+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+
| unused |
+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+
| Internet Header + 64 bits of Original Data Datagram |
+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+
ICMPV4
Type:
#ifndef ICMP_ECHOREPLY
#define ICMP_ECHOREPLY 0 /* Echo Reply */
#endif
#ifndef ICMP_DEST_UNREACH
#define ICMP_DEST_UNREACH 3 /* Destination Unreachable */
#endif
#ifndef ICMP_SOURCE_QUENCH
#define ICMP_SOURCE_QUENCH 4 /* Source Quench */
#endif
#ifndef ICMP_REDIRECT
#define ICMP_REDIRECT 5 /* Redirect (change route) */
#endif
#ifndef ICMP_ECHO
#define ICMP_ECHO 8 /* Echo Request */
#endif
#ifndef ICMP_ROUTERADVERT
#define ICMP_ROUTERADVERT 9
#endif
#ifndef ICMP_ROUTERSOLICIT
#define ICMP_ROUTERSOLICIT 10
#endif
#ifndef ICMP_TIME_EXCEEDED
#define ICMP_TIME_EXCEEDED 11 /* Time Exceeded */
#endif
#ifndef ICMP_PARAMETERPROB
#define ICMP_PARAMETERPROB 12 /* Parameter Problem */
#endif
#ifndef ICMP_TIMESTAMP
#define ICMP_TIMESTAMP 13 /* Timestamp Request */
#endif
#ifndef ICMP_TIMESTAMPREPLY
#define ICMP_TIMESTAMPREPLY 14 /* Timestamp Reply */
#endif
#ifndef ICMP_INFO_REQUEST
#define ICMP_INFO_REQUEST 15 /* Information Request */
#endif
#ifndef ICMP_INFO_REPLY
#define ICMP_INFO_REPLY 16 /* Information Reply */
#endif
#ifndef ICMP_ADDRESS
#define ICMP_ADDRESS 17 /* Address Mask Request */
#endif
#ifndef ICMP_ADDRESSREPLY
#define ICMP_ADDRESSREPLY 18 /* Address Mask Reply */
#endif
#ifndef NR_ICMP_TYPES
#define NR_ICMP_TYPES 18
#endif
Code:
/* Codes for Type3 UNREACH. */
#ifndef ICMP_NET_UNREACH
#define ICMP_NET_UNREACH 0 /* Network Unreachable */
#endif
#ifndef ICMP_HOST_UNREACH
#define ICMP_HOST_UNREACH 1 /* Host Unreachable */
#endif
#ifndef ICMP_PROT_UNREACH
#define ICMP_PROT_UNREACH 2 /* Protocol Unreachable */
#endif
#ifndef ICMP_PORT_UNREACH
#define ICMP_PORT_UNREACH 3 /* Port Unreachable */
#endif
#ifndef ICMP_FRAG_NEEDED
#define ICMP_FRAG_NEEDED 4 /* Fragmentation Needed/DF set */
#endif
#ifndef ICMP_SR_FAILED
#define ICMP_SR_FAILED 5 /* Source Route failed */
#endif
#ifndef ICMP_NET_UNKNOWN
#define ICMP_NET_UNKNOWN 6
#endif
#ifndef ICMP_HOST_UNKNOWN
#define ICMP_HOST_UNKNOWN 7
#endif
#ifndef ICMP_HOST_ISOLATED
#define ICMP_HOST_ISOLATED 8
#endif
#ifndef ICMP_NET_ANO
#define ICMP_NET_ANO 9
#endif
#ifndef ICMP_HOST_ANO
#define ICMP_HOST_ANO 10
#endif
#ifndef ICMP_NET_UNR_TOS
#define ICMP_NET_UNR_TOS 11
#endif
#ifndef ICMP_HOST_UNR_TOS
#define ICMP_HOST_UNR_TOS 12
#endif
#ifndef ICMP_PKT_FILTERED
#define ICMP_PKT_FILTERED 13 /* Packet filtered */
#endif
#ifndef ICMP_PREC_VIOLATION
#define ICMP_PREC_VIOLATION 14 /* Precedence violation */
#endif
#ifndef ICMP_PREC_CUTOFF
#define ICMP_PREC_CUTOFF 15 /* Precedence cut off */
#endif
#ifndef NR_ICMP_UNREACH
#define NR_ICMP_UNREACH 15 /* instead of hardcoding immediate value */
#endif
/* Codes for Type5 REDIRECT. */
#ifndef ICMP_REDIR_NET
#define ICMP_REDIR_NET 0 /* Redirect Net */
#endif
#ifndef ICMP_REDIR_HOST
#define ICMP_REDIR_HOST 1 /* Redirect Host */
#endif
#ifndef ICMP_REDIR_NETTOS
#define ICMP_REDIR_NETTOS 2 /* Redirect Net for TOS */
#endif
#ifndef ICMP_REDIR_HOSTTOS
#define ICMP_REDIR_HOSTTOS 3 /* Redirect Host for TOS */
#endif
/* Codes for Type11 TIME_EXCEEDED. */
#ifndef ICMP_EXC_TTL
#define ICMP_EXC_TTL 0 /* TTL count exceeded */
#endif
#ifndef ICMP_EXC_FRAGTIME
#define ICMP_EXC_FRAGTIME 1 /* Fragment Reass time exceeded */
#endif
ICMPV6
Type:
/** Error Messages: (type <128) */
#define ICMP6_DST_UNREACH 1
#define ICMP6_PACKET_TOO_BIG 2
#define ICMP6_TIME_EXCEEDED 3
#define ICMP6_PARAM_PROB 4
/** Informational Messages (type>=128) */
#define ICMP6_ECHO_REQUEST 128
#define ICMP6_ECHO_REPLY 129
#define MLD_LISTENER_QUERY 130
#define MLD_LISTENER_REPORT 131
#define MLD_LISTENER_REDUCTION 132
#define ND_ROUTER_SOLICIT 133
#define ND_ROUTER_ADVERT 134
#define ND_NEIGHBOR_SOLICIT 135
#define ND_NEIGHBOR_ADVERT 136
#define ND_REDIRECT 137
#define ICMP6_RR 138
#define ICMP6_NI_QUERY 139
#define ICMP6_NI_REPLY 140
#define ND_INVERSE_SOLICIT 141
#define ND_INVERSE_ADVERT 142
#define MLD_V2_LIST_REPORT 143
#define HOME_AGENT_AD_REQUEST 144
#define HOME_AGENT_AD_REPLY 145
#define MOBILE_PREFIX_SOLICIT 146
#define MOBILE_PREFIX_ADVERT 147
#define CERT_PATH_SOLICIT 148
#define CERT_PATH_ADVERT 149
#define ICMP6_MOBILE_EXPERIMENTAL 150
#define MC_ROUTER_ADVERT 151
#define MC_ROUTER_SOLICIT 152
#define MC_ROUTER_TERMINATE 153
#define FMIPV6_MSG 154
#define RPL_CONTROL_MSG 155
#define LOCATOR_UDATE_MSG 156
#define DUPL_ADDR_REQUEST 157
#define DUPL_ADDR_CONFIRM 158
#define MPL_CONTROL_MSG 159
Code:
/** Destination Unreachable Message (type=1) Code: */
#define ICMP6_DST_UNREACH_NOROUTE 0 /* no route to destination */
#define ICMP6_DST_UNREACH_ADMIN 1 /* communication with destination */
/* administratively prohibited */
#define ICMP6_DST_UNREACH_BEYONDSCOPE 2 /* beyond scope of source address */
#define ICMP6_DST_UNREACH_ADDR 3 /* address unreachable */
#define ICMP6_DST_UNREACH_NOPORT 4 /* bad port */
#define ICMP6_DST_UNREACH_FAILEDPOLICY 5 /* Source address failed ingress/egress policy */
#define ICMP6_DST_UNREACH_REJECTROUTE 6 /* Reject route to destination */
/** Time Exceeded Message (type=3) Code: */
#define ICMP6_TIME_EXCEED_TRANSIT 0 /* Hop Limit == 0 in transit */
#define ICMP6_TIME_EXCEED_REASSEMBLY 1 /* Reassembly time out */
/** Parameter Problem Message (type=4) Code: */
#define ICMP6_PARAMPROB_HEADER 0 /* erroneous header field */
#define ICMP6_PARAMPROB_NEXTHEADER 1 /* unrecognized Next Header */
#define ICMP6_PARAMPROB_OPTION 2 /* unrecognized IPv6 option */
ICMP Embedding
- For ICMPv4, when the type is 3–ICMP_DEST_UNREACH, 4–ICMP_SOURCE_QUENCH,5–ICMP_REDIRECT,11–ICMP_TIME_EXCEEDED, 12–ICMP_PARAMETERPROB, ICMPv4 can embed IPv4+UDP/TCP/ICMPv4 to indicate the original message IP, TCP/UDP header information that caused the error.
- Similarly, for ICMPv6, when the type is 1–ICMP6_DST_UNREACH, 2–ICMP6_PACKET_TOO_BIG, 3–ICMP6_TIME_EXCEEDED, 4–ICMP6_PARAM_PROB, it can also embed IPv6+UDP/TCP/ICMPv6 to indicate the original header information that caused the error.
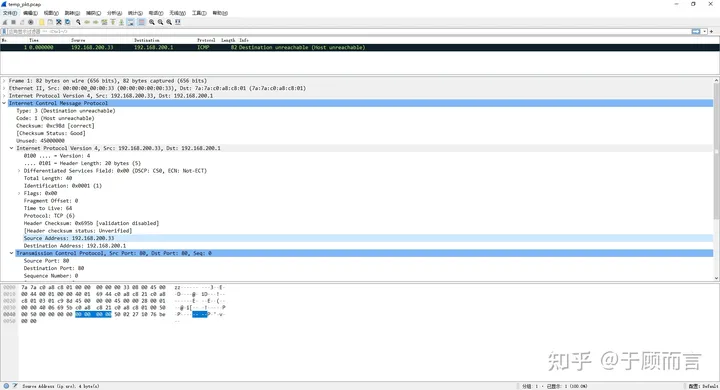
ICMP Attacks
- ICMPv6 Flood
alert icmp any any -> any any (msg:”ET DOS Microsoft Windows 7 ICMPv6 Router Advertisement Flood”; itype:134; icode:0; content:”|03|”; threshold:type threshold, track by_src, count 10, seconds 1; reference:url,http://www.samsclass.info/ipv6/proj/proj8x-124-flood-router.htm; classtype:attempted-dos; sid:2014996; )
- ICMP Smurf Scan
The Smurf attack sets the source IP as the victim’s IP and sends ICMP packets (typically ECHO requests) to multiple servers. These servers, which are fooled by the packets, send ECHO replies (Type=0) back to the victim, resulting in traffic clogging the victim’s network interface.
Here is a rewritten version of the content including the keyword “ICMP alert”:
Alert for ICMP traffic from any external network to any address in the home network. This alert is triggered for an ICMP message with a data size of 4, an ICMP ID of 0, an ICMP sequence of 0, and an ICMP type of 8. This is classified as an attempted reconnaissance with the message “GPL SCAN Broadscan Smurf Scanner.” The alert has a signature ID of 2100478, a revision number of 4, and was created and updated on September 23, 2010.
- ICMP Invalid checksum
alert icmp any any -> any any (msg:”SURICATA ICMPv4 invalid checksum”; icmpv4-csum:invalid; classtype:protocol-command-decode; sid:2200076; rev:2;)
alert icmp any any -> any any (msg:”SURICATA ICMPv6 invalid checksum”; icmpv6-csum:invalid; classtype:protocol-command-decode; sid:2200079; rev:2;)
- ICMP Redirect
This can be exploited for attacks and network eavesdropping. If a certain host A supports ICMP redirect, host B can send an ICMP redirect message to it. Then, all messages from A to the specified address will be forwarded to host B. This allows B to eavesdrop or modify the routing table according to the hacker’s intentions.
alert icmp $EXTERNAL_NET any -> $HOME_NET any (msg:”GPL ICMP_INFO Redirect for TOS and Host”; icode:3; itype:5; classtype:misc-activity; sid:2100436; rev:7; metadata:created_at 2010_09_23, updated_at 2010_09_23;)
Suricata Options Involved in Matching
- itype:[<|>|<>];
Example: This example looks for an ICMP type greater than 10: itype:>10;
2. icode:[<|>|<>];
Example: This example looks for an ICMP code greater than 5: icode:>5;
3. icmp_id:;
Example: This example looks for an ICMP ID of 0: icmp_id:0;
4. icmp_seq:;
Example: This example looks for an ICMP Sequence of 0: icmp_seq:0;
5. icmpv6-csum:<valid/invalid>;
6. icmpv4-csum:<valid/invalid>;